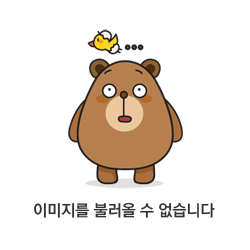
📘 ES6
ES6(ECMAScript 2015)는 JavaScript의 주요 업데이트 중 하나로,
코드의 가독성과 효율성을 높이기 위해 여러 새로운 기능이 추가된 JavaScript 표준이다.
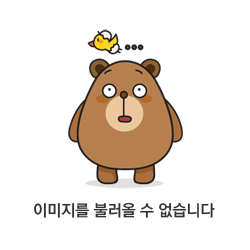
📋 ES6(ECMAScript2015) 주요 변경점
- const, let
- arrow function
- Template Literals
- class
- module
- Destructuring
- Default Parameters
- spread operator & Rest parameter
- Promise
- Symbol
⁉️ const, let
var의 자유로운 사용을 let과 const는 일부 억제함으로 보다 오류 컨트롤이 수월해졌다.
var, let, const 세가지 모두 자바스크립트 엔진이 코드를 실행하기 전 실행컨텍스트를 생성하면서 메모리에 변수 및 함수 선언부를 등록하는 과정 중 호이스팅이 발생하지만, TDZ(Temporal Dead Zone) 이 있어서 선언 전에 변수 혹은 함수를 참조시 참조 에러가 발생해 런터임 에러를 최소화 할 수 있고, 가독성을 높일 수 있다.
// declaration & initialization state output
var a; // undefined
let b; // undefined
const c; // error const must be initialized immediately after declaration.
//=====================================================================//
// hoisting
console.log(a); // undefined
console.log(b); // ReferenceError : TDZ
console.log(c); // ReferenceError : TDZ
var a = 5;
let b = 4;
const c = 3;
//=====================================================================//
// redeclaration
var a = 5;
let b = 4;
const c = 3;
var a = 'test1'; // a = 'test1'
let b = 'test2'; // SyntaxError
const c = 'test3'; // SyntaxError
//=====================================================================//
// reinitialization
var a = 5;
let b = 4;
const c = 3;
a = 'test1'; // a = 'test1'
b = 'test2'; // b = 'test2'
c = 'test3'; // TypeError: Assignment to constant variable.
⁉️ arrow function
- 가독성이 좋아졌다. (기존 함수보다 간략)
- 콜백 함수 내부에서 this가 전역 객체를 가리키는 문제를 해결하기 위한 대안으로 등장
→ 콜백 함수 내에서 this를 직접 바인딩할 필요가 줄어 들었다.
const add (num1,num2) => {
return num1 + num2;
}
// 한줄로 함수 표현이 가능한 경우 아래와 같이 return 을 생략해도 암묵적으로 return이 이루어짐
// 단, 표현식인 경우 한 줄로 표현 할 수 없고 중괄호를 사용해함.
// const add(num1,num2) => num1 + num2;
const result = add(1,2);
console.log(result); // output: 3
⁉️ Template Literals (``)
백틱(`)을 사용하여 문자열 내에 변수를 쉽게 삽입할 수 있게되었다.
const name = "Alice";
console.log(`Hello, ${name}!`); // output: Hello, Alice
⁉️ class
- class 문법은 문법적 설탕(syntactic sugar)이며, 기존의 프로토타입을 기반으로 하지만
더욱 직관적이고 깔끔하게 코드를 작성할 수 있게 해준다. - 기본적으로 public이나 ES2019(ES10) 이후 private(’#’으로 표현)이 추가되어 사용가능해졌다.
- static 사용 가능 → static 사용시 className.staticFunction() 형식으로 호출 생성자함수로 변수에 클래스가 할당되더라도
변수.staticFunc() 이 아닌 className.staticFunction() 형식으로만 사용 가능하다.
class Student {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHi() {
return `Hi! ${this.name} Are you ${this.age} years old?`;
}
}
class School extends Student {
constructor(name, age, classNum) {
super(name, age);
this.classNum = classNum;
}
sayHi() {
return `${super.sayHi()} And ${this.classNum} is your class number?`;
}
}
const student = new School('Lee', 24, 4);
console.log(student.sayHi()); // output: Hi! Lee Are you 24 years old? And 4 is your class number?
⁉️ module (require → export / import)
코드 재사용성이 증가하고, 명확한 종속성 관리가 가능해졌다.
// math.js
export const add = (a, b) => a + b;
// main.js
import { add } from './math';
console.log(add(2, 3)); // 5
⁉️ Destructuring
코드가 간결해지며, 변수에 값을 할당할 때 유용하게 사용할 수 있다.
const [x, y] = [1, 2];
const { name, age } = { name: "Alice", age: 25 };
⁉️ Default Parameters
함수 호출 시 인자가 전달되지 않을 때 기본값을 지정할 수 있어 코드가 간결해졌다.
function greet(name = "Guest") {
console.log(`Hello, ${name}`);
}
greet(); // output: Hello, Guest
// greet.length => 0
⁉️ spread operator & Rest Parameter
배열 복사, 객체 병합 등이 쉬워졌고, 함수 인자 처리도 유연해졌다.
이론 정리 바로 가기 [https://ama-grammer.tistory.com/43]
const arr = [1, 2, 3];
const newArr = [...arr, 4, 5];
function sum(...nums) {
return nums.reduce((acc, cur) => acc + cur, 0);
}
const result = sum(...newArr);
console.log(result) // output: 15
⁉️ Promise
비동기 작업을 체인 형식으로 처리할 수 있어 코드가 읽기 쉬워지고, 에러 처리가 간편해졌다.
const fetchData = () => new Promise((resolve, reject) => {
setTimeout(() => resolve("Data loaded"), 1000);
});
fetchData().then(data => console.log(data)); // 1000ms 후 output: Data loaded
⁉️ Symbol
객체의 프로퍼티 키를 유일하게 정의할 수 있어, 프로퍼티 이름의 충돌을 방지할 수 있다.
const id = Symbol("id");
const user = {
[id]: 123,
name: "Alice"
};
🔥 배운점
Modern JavaScript 를 학습하며 가장 큰 변화가 있었던 ES6 의 내용을 정리했다.
위에 정리된 내용 이외에도 다양한 변경점이 있지만, 그중 몇개를 골라 정리해보았고 정리를 하니 일부 까먹은 내용들도 복습할 수 있었다.
💡 참고 사이트
https://www.w3schools.com/js/js_es6.asp
W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
'기술면접 개념 정리' 카테고리의 다른 글
[기술면접] JWT (0) | 2024.11.25 |
---|---|
[기술면접] 브라우저 저장소 개념정리 (1) | 2024.11.19 |
[기술면접] Webpack & Babel (0) | 2024.11.14 |
[기술면접] SSR vs CSR (2) | 2024.10.28 |